EC6611 COMPUTER NETWORKS (CN) Lab Manual. Study of Socket Programming and Client – Server model; Write a socket Program for Echo/Ping/Talk commands. To create scenario and study the performance of network with CSMA / CA protocoland compare with CSMA/CD protocols. I'm writing my first java client/server program which just establishes a connection with the server sends it a sentence and the server sends the sentence back all capitalized.
I have a TCP Client which continuously sends data to the server(The server is running continuously). It creates connection each time to the server to send the data. However, it throws exception after sending some amount of data to the server. Data is coming from some sensors continuously and that data is sent by my TCP Client to the server.
Here is my piece of TCPClient code :
try {
System.out.println('Inside Client :' + device_data);
logger.info('Inside Client :' + device_data);
InputStream is = new ByteArrayInputStream(device_data.getBytes());
BufferedReader socketRead = new BufferedReader(new InputStreamReader(is));
/**
* sending the contents to server. Uses PrintWriter
*/
OutputStream ostream = sock.getOutputStream();
PrintWriter pwrite = new PrintWriter(ostream, true);
String str;
/**
* Reading data line by line
*/
while ((str = socketRead.readLine()) != null) {
if(!pwrite.checkError()){
pwrite.println('Data sent by Client : ' + str);
logger.info('Data sent by client' + str);
System.out.println('Data sent by client' + str);
}
else{
logger.info('Error in writing data');
System.out.println('Error in writing data');
}
}
pwrite.close();
socketRead.close();
}
catch (Exception exception) {
System.out.println('Exception' + exception);
logger.info('Exception in TCPClient :' + exception);
}
Thanks in advance.
Hi I need to execute the PING
command using Java code and get summary of the ping host. How to do it in Java?
4 Answers
as viralpatel specified you can use Runtime.exec()
following is an example of it
output
refer http://www.velocityreviews.com/forums/t146589-ping-class-java.html
Uwe PlonusInetAddress
class has a method which uses ECMP Echo Request (aka ping) to determine the hosts availability.
If the above reachable
variable is true, then it means that the host has properly answered with ECMP Echo Reply (aka pong) within given time (in millis).
Note: Not all implementations have to use ping. The documentation states that:
A typical implementation will use ICMP ECHO REQUESTs if the privilege can be obtained, otherwise it will try to establish a TCP connection on port 7 (Echo) of the destination host.
Therefore the method can be used to check hosts availability, but it can not universally be used to check for ping-based checks.
DariuszDariuszCheck out this ping library for java I'm making:
Maybe it can help
tgoossenstgoossensSocket Program For One Way Communication
Below code will work on both Windows and Linux system,
Rakesh ChaudhariRakesh ChaudhariWrite A Socket Program For Echo/ping/talk Commands In Java
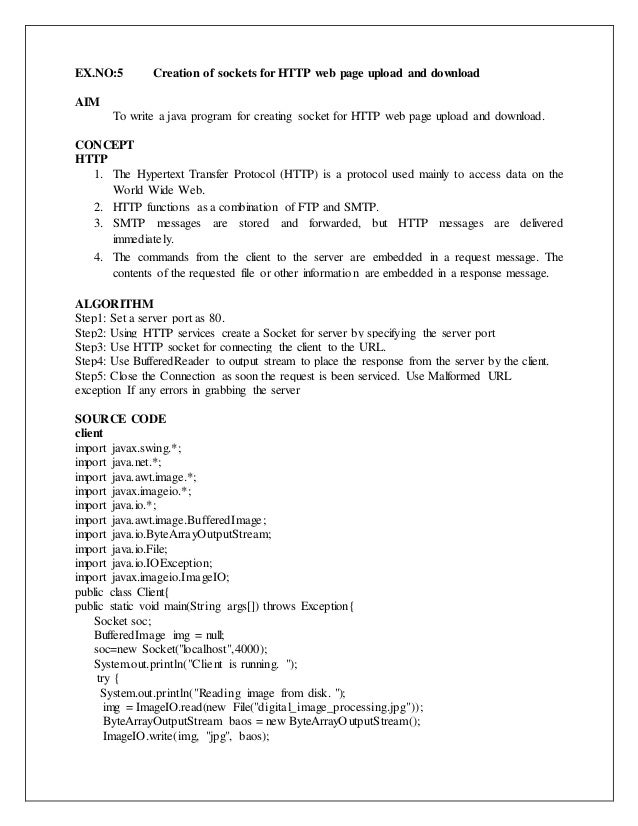
Comments are closed.